Prototyping a project using Google Apps Script
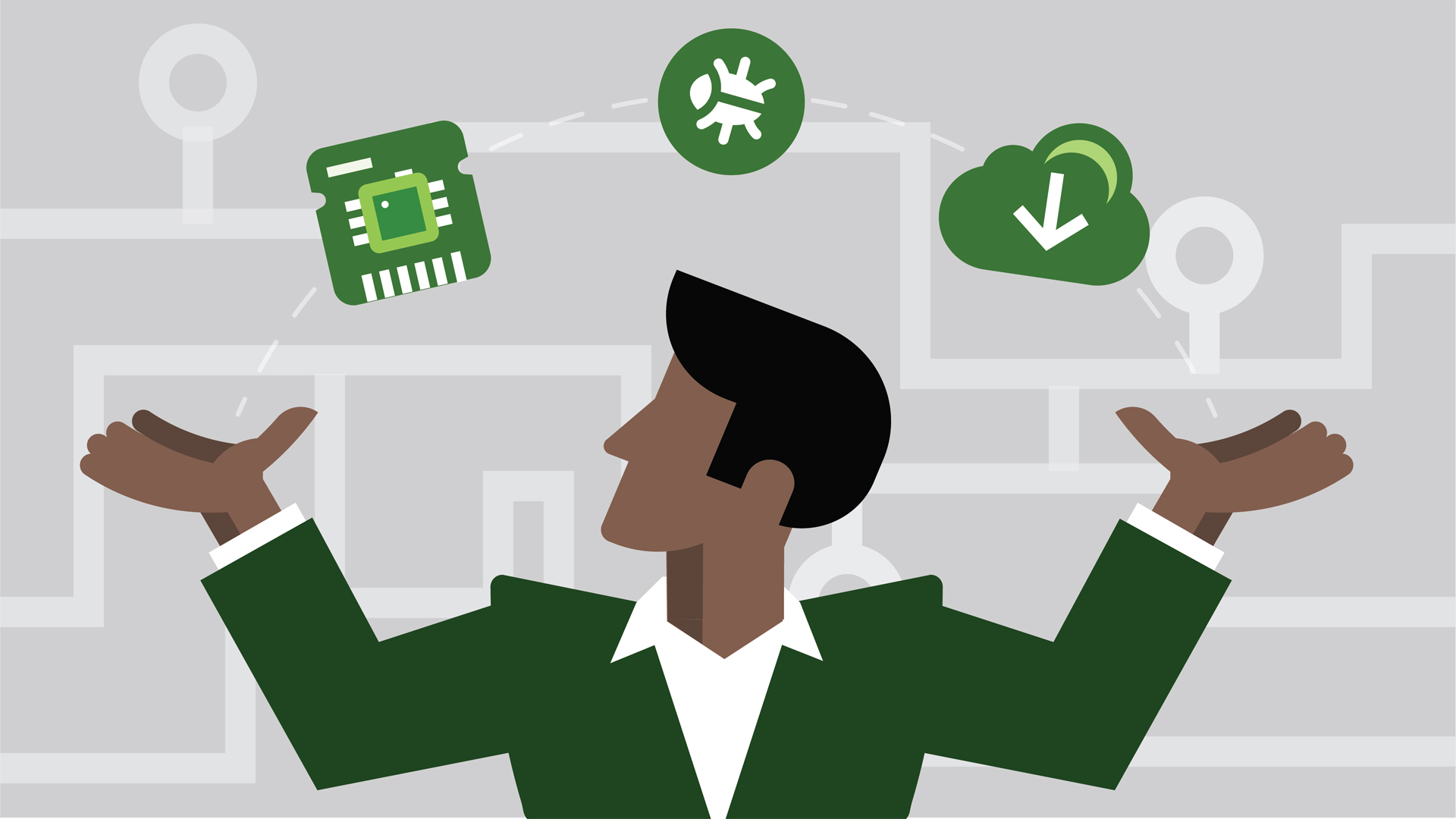
Prototyping a project for a client using Google Apps Script, I ran into a curious aspect of Google Drive, that allows the same file name to be used within the same folder. In addition the same file name can be spread across multiple folders. Exploring how to get detailed information on a file, I used the prototype to gather information on each file; such as full parent folder path, file URL and file ID.
I created the following folder structure to test my prototype.
My Drive
--|Test00
--|Test02
--|Test03
I started with a simple main() function to call the primary worker function and log my returned results. We will search for a file named 'Test01'.
function main() {
var fileInfo = getFileInfo("Test01");
Logger.log(fileInfo);
};
The getFileInfo() function uses the 'DriveApp' class, which allows scripts to create, find, and modify files and folders in Google Drive. The call to 'DriveApp.getFilesByName(fileName)' gets a collection of files in the user's Drive that have the given name by returning 'FileIterator'. Now we can iterate the files returned and proceed to gather more information on each file.
function getFileInfo(fileName) {
var files = DriveApp.getFilesByName(fileName);
var filesJObj = {"files":[]};
while(files.hasNext()) {
var file = files.next();
var fileName = file.getName();
var fileURL = file.getUrl();
var fileID = file.getId();
var parentFolder = getParentFolder(file);
var jobjFileID = { "file":fileName, "ID": fileID, "folder":
parentFolder, "URL": fileURL};
filesJObj['files'].push( jobjFileID );
};
return(filesJObj);
};
Most of the file information is a simple function call to retrieve. Getting the file-name is called by
'file.getName();'
Getting the file-URL is called by 'file.getUrl();'
Getting the file-ID is called by 'file.getId();'
Last piece of the puzzle is creating a folder structure per file location. This will return a full path to a file that looks like this:
“/My Drive/Test00/Test02/”
function getParentFolder(file) {
var parentFolder = "";
var parents = file.getParents();
while (parents.hasNext()) {
var folder = parents.next();
parentFolder = folder.getName() + "/" + parentFolder ;
parents = folder.getParents();
}
parentFolder = "/" + parentFolder;
return(parentFolder);
};
Putting it all together, allows you to gather more information per file and extend the template to your needs.
Full Code Example:
--------------------------------------------------------------
function getParentFolder(file) {
var parentFolder = "";
var parents = file.getParents();
while (parents.hasNext()) {
var folder = parents.next();
parentFolder = folder.getName() + "/" + parentFolder ;
parents = folder.getParents();
}
parentFolder = "/" + parentFolder;
return(parentFolder);
};
function getFileInfo(fileName) {
var files = DriveApp.getFilesByName(fileName);
var filesJObj = {"files":[]};
while(files.hasNext()) {
var file = files.next();
var fileName = file.getName();
var fileURL = file.getUrl();
var fileID = file.getId();
var parentFolder = getParentFolder(file);
var jobjFileID = { "file":fileName, "ID": fileID, "folder":
parentFolder, "URL": fileURL};
filesJObj['files'].push( jobjFileID );
};
return(filesJObj);
};
function main() {
var fileInfo = getFileInfo("Test01");
Logger.log(fileInfo);
};
--------------------------------------------------------------
Log Output:
{files=[
{file=Test01, ID=1tv3mn8UL5_4FqRNQKwzpgbPGdyhj-Vahn4_ovUZpq9A,
URL=https://docs.google.com/document/d/1tv3mn8UL5_4FqRNQKwzpgbPGdyhj-Vahn4_ovUZpq9A/edit?usp=drivesdk, folder=/My Drive/Test00/},
{ID=133ai1b06S4xinzXSlVgcKovJTZ5WdkXVnkq72GTR4L8, folder=/My Drive/Test00/, file=Test01, URL=https://docs.google.com/spreadsheets/d/133ai1b06S4xinzXSlVgcKovJTZ5WdkXVnkq72GTR4L8/edit?usp=drivesdk},
{file=Test01, ID=1i8FHBj3v0R3HkX4e4YVVTjGwjjiWdsW2CKWLpthqk64, URL=https://docs.google.com/document/d/1i8FHBj3v0R3HkX4e4YVVTjGwjjiWdsW2CKWLpthqk64/edit?usp=drivesdk, folder=/My Drive/Test00/Test02/},
{folder=/My Drive/Test00/Test03/, ID=1QNYVfo0S-QG1JrMBSavoUyOCRypInS_oUaNId6Q_2-o, file=Test01, URL=https://docs.google.com/document/d/1QNYVfo0S-QG1JrMBSavoUyOCRypInS_oUaNId6Q_2-o/edit?usp=drivesdk}]}