Abstraction in Java
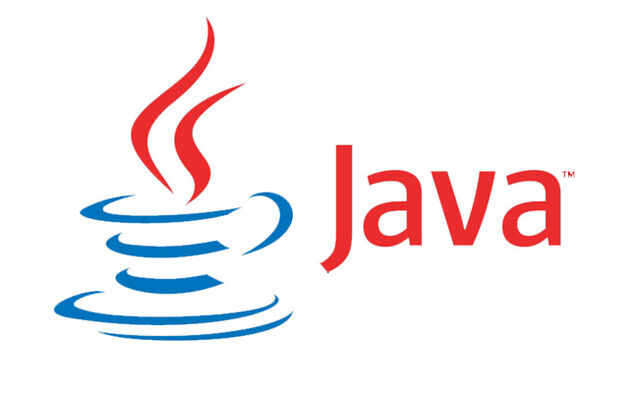
Abstraction is dealing with ideas rather than events. For example, when we consider e-mail, complex details such as what happens as soon as you send an e-mail, the protocol your e-mail server uses are hidden from the user. Therefore, to send an e-mail we just need to type the content, mention the address of the receiver, and click send. In Object-oriented programming, abstraction is a process of hiding the implementation details from the user, only the functionality will be provided to the user. In other words, the user will have the information on what the object does instead of how it does it. Java uses abstract classes and interfaces to achieve abstraction.
Abstract class
In Java, we declare abstract classes using the abstract keyword. Abstract classes can have methods without bodies like interfaces, which we will see later. If a class does have at least one abstract method, the class must be declared abstract. Abstract classes can't be instantiated. In order to Use abstract classes, we have to inherit it from another class and have implementation to the abstract methods.
1 public abstract class Student 2 { 3 private String name; 4 private String grade; 5 private double score; 6 7 public Student(String p_name, String p_grade, double p_score) 8 { 9 System.out.println("Constructing a Student"); 10 this.name = p_name; 11 this.grade = p_grade; 12 this.score = p_score; 13 } 14 15 public double computeScore( ) 16 { 17 System.outprintln ( " We are in computeScore"); 18 } 19 20 public String getName() 21 { 22 return name; 23 } 24 25 public String getGrade() 26 { 27 return grade; 28 } 29 30 }
Using the abstract class
We can't instantiate an abstract class but we can create another class to inherit the abstract class. We can then instantiate the new class. To inherit an abstract class, we use the keyword extends. After we inherit the abstract class, we then have to fill the details of the abstract methods in the abstract class. Once that is done, we can instance the new class. In the example below, we will inherit the abstract class from the example above and instance the new class.
public class Chemistry extends Student { double currAvg ; public Chemistry(String p_name, String p_grade, double p_score, double p_currAvg) { super(p_name, p_grade, p_score) ; setAvg( currAvg ); } public double computeScore { System.out.println("Within mailCheck of Salary class "); System.out.println("Mailing check to " + getName() + " with salary " + salary); } public void setAvg( double p_currAvg ) { currAvg = p_currAvg ; } public double computeScore() { return currAvg - score ; } } public class MyExample { public static void main(String [] args) { Chemistry subject = new Chemistry("John Doe", "Senior", 98.5, 95.6); if ( subject.computeScore > 0 ) { System.out.println("Chemistry is bringing your average up"); } else { System.out.println("Chemistry is bringing your average down"); } } }
Abstract methods
If we want a class to contain a method but we want the actual implementation of that method to be determined by child classes, we can declare the method in the parent class as an abstract. Just like the abstract class, an abstract method is declared using the abstract keyword. An abstract method has a method signature but no method body. Instead of curly braces, an abstract method ends with a semicolon. As we discussed earlier, if we use an abstract method in a class, the class must be declared abstract. Also any class that inherits the class must either override the method or be declared an abstract method itself.
public abstract class Student { private String name; private String grade; private double score; public abstract double computeScore(); }