Interfaces in Java
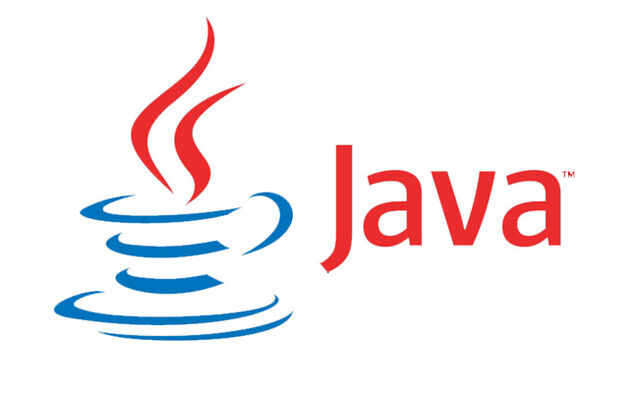
An interface is a reference type in Java. It is similar to class. It is a collection of methods without any code. A class implements an interface, thereby inheriting the abstract methods of the interface. Along with abstract methods, an interface may also contain constants, default methods, static methods, and nested types. Method bodies exist only for default methods and static methods. Writing an interface is similar to writing a class except a class describes the attributes and behaviors of an object and an interface contains behaviors that a class implements. Unless the class that implements the interface is abstract, all the methods of the interface need to be defined in the class.
An interface is similar to a class. An interface can contain any number of methods. An interface is written in a file with a .java extension, with the name of the interface matching the name of the file. The byte code of an interface appears in a .class file. Interfaces appear in packages, and their corresponding bytecode file must be in a directory structure that matches the package name.
An interface is different from a class in several ways. W can't instantiate an interface. An interface does not contain any constructors. All of the methods in an interface are abstract. An interface cannot contain instance fields. The only fields that can appear in an interface must be declared both static and final. An interface is not extended by a class, it is implemented by a class. An interface can extend multiple interfaces.
Declaring
The interface keyword is used to declare an interface. An interface is implicitly abstract, we don't need to use the abstract keyword while declaring an interface. Each method in an interface is also implicitly abstract, so the abstract keyword is not needed. Methods in an interface are implicitly public.
Example of an interface declaration
interface Animal { public void eat(); public void sleep(); public void procreate() ; }
Implementing interfaces
When a class implements an interface, we can think of the class as signing a contract, agreeing to perform the specific behaviors of the interface. If a class does not perform all the behaviors of the interface, the class must declare itself as abstract. A class uses the implements keyword to implement an interface. The implements keyword appears in the class declaration following the extends portion of the declaration. A class can implement more than one interface at a time. A class can extend only one class, but implement many interfaces. An interface can extend another interface, in a similar way as a class can extend another class.
public class HumanInt implements Animal { public void eat() { System.out.println("Human eats"); } public void sleep() { System.out.println("Humans sleeps"); } public void procreate() { System.out.println("Humans have children"); } public static void main(String args[]) { HumanInt man = new HumanInt(); man.eat(); man.sleeps(); } }
Extending interfaces
An interface can extend another interface in the same way that a class can extend another class. The extends keyword is used to extend an interface, and the child interface inherits the methods of the parent interface. A Java class can only extend one parent class, multiple inheritance is not allowed. Since interfaces are not classes, an interface can extend more than one parent interface. The extends keyword is used once, and the parent interfaces are declared in a comma-separated list.
public interface Sports { public void setHome(String name); public void setVisitor(String name); } public interface Football extends Sports { public void homeScored(int points); public void endQuarter(int quarter); }
Any method that implements Football must define 4 methods, two from the Sports interface and two from the Football interface.