Input / Output in Java
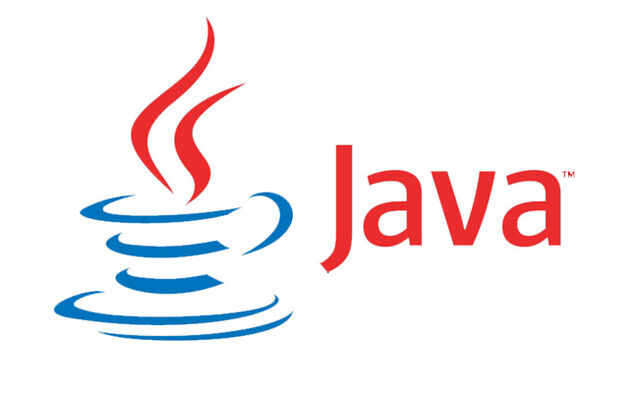
Java IO is an API that comes with Java which is targeted at reading and writing data. Most applications need to process some input and produce some output based on that input. For example, read data from a file or over network, and write to a file or write a response back over the network. The Java IO API is located in the Java IO package java.io. The java.io package doesn't cover all types of input and output. For example, input from and output to a GUI or web page is not covered in the Java IO package.
The Java IO package is focused on input and output to files, network streams, internal memory buffers etc. Java does this mainly with streams. Streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, objects, and localized characters. A stream can be defined as a sequence of data. There are two kinds of Streams. The first stream is the InPutStream which is used to read data from a source. The next is the OutPutStream which is used for writing data to a destination. In this tutorial, we will cover the basic stream types.
Byte Stream
Java byte streams are used to perform input and output of 8-bit bytes. Though there are many classes related to byte streams but the most frequently used classes are, FileInputStream and FileOutputStream.
import java.io.*; public class CopyFile { public static void main(String args[]) throws IOException { FileInputStream in = null; FileOutputStream out = null; try { in = new FileInputStream("input.txt"); out = new FileOutputStream("output.txt"); int x; while ((x = in.read()) != -1) { out.write(x); } } finally { if (in != null) { in.close(); } if (out != null) { out.close(); } } } }
Character streams
Java Character streams are used to perform input and output for 16-bit unicode. This is different from the byte stream above which dies 8-bit bytes. Though there are many classes related to character streams but the most frequently used classes are, FileReader and FileWriter. Internally, FileReader uses FileInputStream and FileWriter uses FileOutputStream but the difference is that FileReader reads two bytes at a time and FileWriter writes two bytes at a time.
import java.io.*; public class CopyFile { public static void main(String args[]) throws IOException { FileReader in = null; FileWriter out = null; try { in = new FileReader("input.txt"); out = new FileWriter("output.txt"); int x; while ((x = in.read()) != -1) { out.write(c); } } finally { if (in != null) { in.close(); } if (out != null) { out.close(); } } } }
Standard streams
All programming languages provide support for standard I/O where the program can take input from a keyboard and then produce an output on the computer screen. In C or C++ programming languages, there are three standard devices STDIN, STDOUT and STDERR. Similarly, Java provides three streams also. The standard input, or STDIN in C, is usually the keyboard. In Java, we call it system.in. The standard output, or STDOUT in C, is usually the monitor. In Java, we call it system.out. The standard error, or STDERR in C, is used to output the error to a monitor. In Java, we call it system.err.
import java.io.*; public class ReadChars { public static void main(String args[]) throws IOException { InputStreamReader cinput = null; try { cInput = new InputStreamReader(System.in); System.out.println("Enter characters, 'q' to quit."); char singleChar; do { singlechar = (char) cinput.read(); System.out.print(singleChar); } while(singleChar != 'q'); } finally { if (cinput != null) { cinput.close(); } } } }