Access modifiers in Java
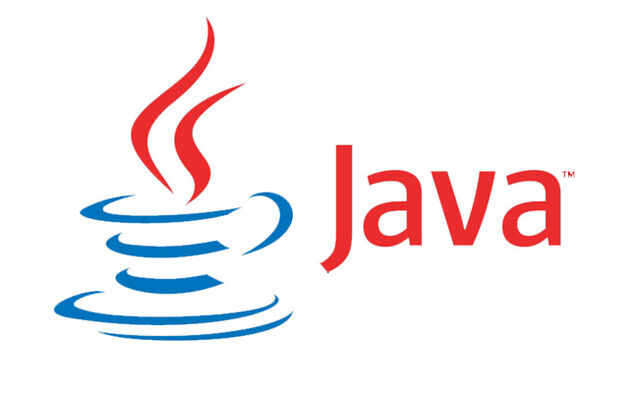
Java provides access modifiers to set access levels for classes, variables, methods, and constructors. There are four levels of access in Java. The first is visible to he package and is the default, no modifiers are needed. The second is visible to the class only and uses the private keyword. The third is visible everywhere and uses the public keyword. The fourth is visible to the package and all subclasses and uses the protected keyword. As the name implies, access modifiers in Java helps to restrict the scope of a class, constructor, variable, method or data member. If other programmers use your class, try to use the most restrictive access level that makes sense for a particular member. Use private unless you have a good reason not to. Avoid public fields except for constants.
private default protected public Class No Yes No Yes Nested Class Yes Yes Yes Yes Constructor Yes Yes Yes Yes Method Yes Yes Yes Yes Field Yes Yes Yes Yes
Default, no keyword
When we don't use any keyword explicitly, Java will set a default access to a given class, method or property. The default access modifier is also called package-private, which means that all members are visible within the same package but aren’t accessible from other packages. Subclasses cannot access methods and member variables (fields) in the superclass, if they these methods and fields are marked with the default access modifier, unless the subclass is located in the same package as the superclass.
class MyClass { void myMethod() { System.out.println("Hello World!"); } }
Private
Methods, variables, and constructors that are declared private can only be accessed within the declared class. Private access modifier is the most restrictive access level. Class and interfaces cannot be private. Variables that are declared private can be accessed outside the class, if public getter methods are present in the class. Using the private modifier is a way for an object to encapsulate itself and hides data from the outside world. Classes cannot be marked with the private access modifier. Marking a class with the private access modifier would mean that no other class could access it, which means that we can't use the class at all. The private access modifier is not allowed for classes.
class MyClass { private int myNumber = 15 ; }
Public
A class, method, constructor, or interface that is declared public can be accessed from any other class. Fields, methods, blocks declared inside a public class can be accessed from any class. If the public class we are trying to access is in a different package, then the public class still needs to be imported. Because of class inheritance, all public methods and variables of a class are inherited by its subclasses. This is the least restrictive access modifier.
public static void main(String[] arguments) { System.out.println("Hello World!"); }
Protected
Variables, methods, and constructors, that are declared protected in a superclass can be accessed only by the subclasses in other package or any class within the package of the protected members' class. The protected access modifier cannot be applied to class and interfaces. Methods, fields can be declared protected, but methods and fields in a interface cannot be declared protected. Protected access gives the subclass access to the helper method or variable, while preventing a non-related class from trying to use it. Essentially, the protected access modifier provides the same access as the default access modifier, with the addition that subclasses can access protected methods and member variables of the superclass. This is true even if the subclass is not located in the same package as the superclass.
class MyClass { protected void hello() { System.out.println("Hello World"); } } class MyClass2 extends MyClass { public static void main(String args[]) { mMyClass2 myObj = new MyClass2(); myObj.hello(); } }