Control statements - decision making statements in Java
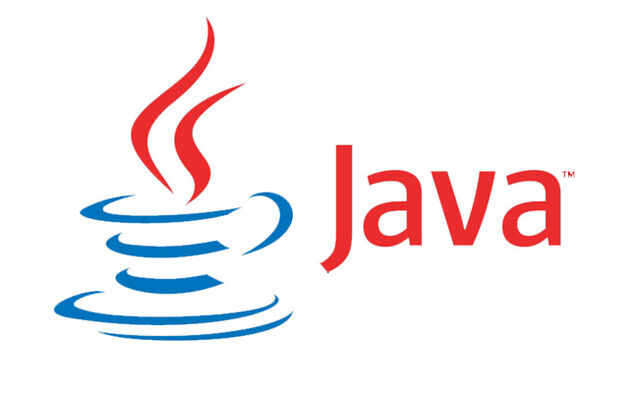
Control structures can be grouped into three categories: decision making statements, iteration statements and branching statements. Decision making statements are used to decide whether or not a particular statement or a group of statements should be executed. Iteration statements are used to execute a statement or a group of statements more than once. Branching statements are used to transfer control to another line in the code. Decision making statements allow us to control the flow of program execution based the outcome of an expression or state of a variable known during runtime. Decision making statements consists of if, if then else, and switch. We will explore decision making statements in this article.
If and if else statement
The if statement is used to selectively execute a statement or a block of statements. The if statement contains a condition which decides whether or not the statements would be executed. If the condition stated in the parentheses evaluates to true, then the statements are executed, otherwise the statements in the if block are skipped. The if statement is known as a single selection structure since we have only a single statement that is either executed or not. No alternative statement would be executed if the condition evaluates to false. In order to check for an alternative condition, we can use the if else double selection structure.
1 public class IfDemo 2 { 3 public static void main(String[] args) 4 { 5 6 int age; 7 age = 19 ; 8 if(age > 18) 9 { 10 System.out.println("above 18 "); 11 } 12 13 if ( age >= 18 ) 14 { 15 System.out.println( "You are an adult " ); 16 } 17 else 18 { 19 System.out.println( "You are a minor" ); 20 } 21 22 if ( age >= 65 ) 13 { 24 System.out.println( "You are a senior citizen " ); 25 } 26 else if ( age >= 18 && age < 65 ) 27 { 28 System.out.println( "You are an adult" ); 29 } 30 else 31 { 32 System.out.println( "You are a minor" ); 33 } 34 } 35 }
Switch statements
The switch statement in Java is another decision making statement that defines multiple paths of execution of a program. The switch statement is a multi way branch statement. It provides a better alternative than a large series of if-else-if statements. Unlike if-else-if statements, the switch control structure can't be used when the condition needs to contain relational operators like greater than, less than and so on. The switch structure can only be used to check for equality. The data types that can be used as a part of switch expression are byte, short, int, char, and String. Strings are checked for equality by using the equals() method and not by using the == operator which is the used for the other data types. If no match is found, the statements under the default label are executed. The break keyword is used to transfer control outside the switch block. If the break keyword is omitted, the statements under the other case labels are also executed whether or not the case value matches. All the case values should be unique.
1 public class IfDemo 2 { 3 public static void main(String[] args) 4 { 5 6 int age; 7 age = 19 ; 8 switch ( age ) 9 { 10 case 1: 11 case 2: 12 case 3: 13 case 4: 14 case 5: 15 case 6: 16 case 7: 17 case 8: 18 case 9: 19 case 10: 20 case 11: 21 case 12: 22 case 13: 23 case 14: 24 case 15: 25 case 16: 26 case 17: 27 case 18: 28 case 19: 29 case 20: 30 case 21: 31 System.out.println( "you can't drink" ); 32 break; 33 case 30: 34 System.out.println( "you are 30" ); 35 break; 36 default: 37 System.out.println( "You are not 30 nor can you drink" ); 38 break; 39 } 40 }
Lines 10 - 30 all execute the code in line 31.