Object basics in JavaScript part 2
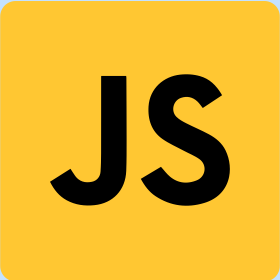
Continued from Object basics in JavaScript part 1.
Comparison by reference
The equality == and strict equality === operators for objects work exactly the same as for primitive data types. Two objects are equal only if they are the same object. For example, if two variables reference the same object, they are equal. Two independent objects are not equal, even though both are empty or contain the same information since they reference different objects. For a comparison between object or against a primitive such as obj == 5, objects are converted to primitives. Comparisons between objects and other objects or primitives are rare and are usually coding errors.
1 let a = {}; 2 let b = a; 3 let c = {}; 4 let d = 5 alert( a == b ); 6 alert( a === b ); 7 alert( a == c );
Lines 5 and produces true since both a and b reference the same object. Line 7 produces false since they reference two different objects.
Cloning an object
Copying an object variable creates one more reference to the same object. If we need to duplicate an object, it is a little more involved because there's no built-in method for that in JavaScript. We need to create a new object and replicate the structure of the existing one by iterating over its properties and copying them on the primitive level. We can also use the built in JavaScript method Object.assign( ) to copy objects as well as merging existing objects into a new object. When using the Object.assign() method, be careful because if the receiving object has the same property name, it will be overwritten and if the type does not match, will result in a TypeError.
1 let user = 2 { 3 name: "John", 4 age: 30 5 }; 6 7 let userCopy = {}; 8 let userCopy2 = {}; 9 10 for (let key in user) 11 { 12 userCopy[key] = user[key]; 13 } 14 15 Object.assign( userCopy2, user ); 16 17 let someObj = {name: "foo" } ; 18 let address = {addr: "1 any street" }; 19 Object.assign( someObj, address ) ;
Lines 10 - 13 will iterate through all the properties of the user object and copy it to the userCopy object. After executing line 15, userCopy2 will have the same properties and values as user. After executing line 19, the object someObj will have the properties name and addr with their corresponding values.