Object basics in JavaScript part 1
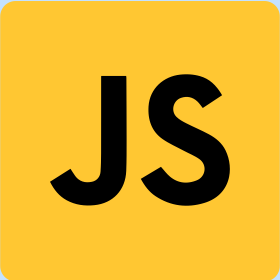
There are seven data types in JavaScript. Six of them are primitive, because their values contain only a single thing whether it is a string or a number or whatever. Objects, on the other hand, are used to store keyed collections of various data and more complex entities. In JavaScript, objects are used in almost every aspect of the language. So we must understand them first before doing anywhere else. An object can be created with curly brackets {…} with an optional list of properties. A property is a key: value pair, where key is a string also called a property name, and value can be anything. We can think of an object as a box with signed files. Every piece of data is stored in its file by the key. It’s easy to find a file by its name or add/remove a file.
Literals and properties
An empty object can be created using one of two syntaxes:
let user = new Object();
let user = {};
The above declaration is called and object literal. We can immediately put some properties between the curly braces as key / value pairs. We can add, remove or access properties from the object at any time. Property values are accessed using the dot notation or for multiword properties, use the square bracket notation object["PROPERTY NAME"].
1 alert( user.name ) ; 2 user.alive = true ; 3 delete user.age ; 4 alert( user["first name"] ) ;
Line 1 accesses the property, Line 2 adds a property. Line 3 deletes a property and line 4 accesses a multiword property.
Copying by reference
One of the fundamental differences of objects vs primitives is that they are stored and copied by reference. Primitive values: strings, numbers, booleans; are assigned / copied as a value. A variable stores not the object itself, but its address in memory, in other words a reference to it. When an object variable is copied, the reference is copied, the object is not duplicated.
Object basics in JavaScript part 2