Functions in JavaScript
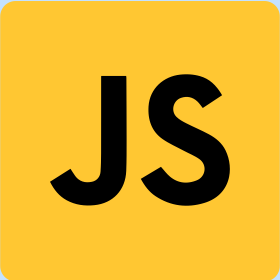
Many times, we need to perform a similar action in many places of the script. For example, we need to show a message when a visitor logs in, logs out and maybe somewhere else. Functions are the main building blocks of the program. They allow the code to be called many times without repetition. JavaScript provides many built in functions like alert(message) and prompt(message, default). We can create functions of our own as well. To create a function we can use a function declaration. The function keyword goes first, then goes the name of the function, then a list of parameters ( if any ) between the parentheses and finally the code of the function between curly braces. Below is an example of a function declaration :
function showMessage() { alert( 'Hello World' ); }
Variables
JavaScript has two types of variables, outer and inner. The inner variables are declared in the function body and only accessible within the function and the outer variables are declared outside the function declaration but still available to the function. You can learn more about variable scope here. If the same name is used for an outer and inner variable, the variable can be modified inside the function, to prevent this, we can use the let keyword inside the function.
let userName = "John" ; function Msg1() { userName = "Bob" ; } function Msg2() { let userName = "Jane" ; } alert(userName) ; Msg1() ; alert(userName) ; Msg2() ; alert(userName) ;
The above code will output John , Bob and Jane. Note that Msg1 changed the variable userName since we didn't use the let keyword while Msg2 did not.
Passing parameters
We can pass parameters into the function by listing them between the parenthesis. The number of parameters expected must match the number of parameters passed. If we don't pass the same number of parameters as expected, we can also have optional parameters by setting a default value for a parameter. Be careful to put the optional parameters at the end of the parameter list. In JavaScript, a default parameter is evaluated every time the function is called without the respective parameter. This is different from some other languages like Python, where any default parameters are evaluated only once during the initial interpretation.
function Msg1( p_name, p_greeting ) { let msg = greeting + " " + p_name ; } function Msg2( p_name, p_greeting = "Hello" ) { let msg = greeting + " " + p_name ; }
Return values
A function can return a value back into the calling code as the result. The directive return can be in any place of the function. When the execution reaches it, the function stops, and the value is returned to the calling code. There may be many occurrences of return in a single function although this is considered bad programming practice. If a function does not return a value, it is the same as if it returns undefined.
function sum(a, b) { return a + b; } function checkVotingAge(age) { if (age > 18) { return true; } else { return false; } } function Msg1() { return ; }