Functions in C
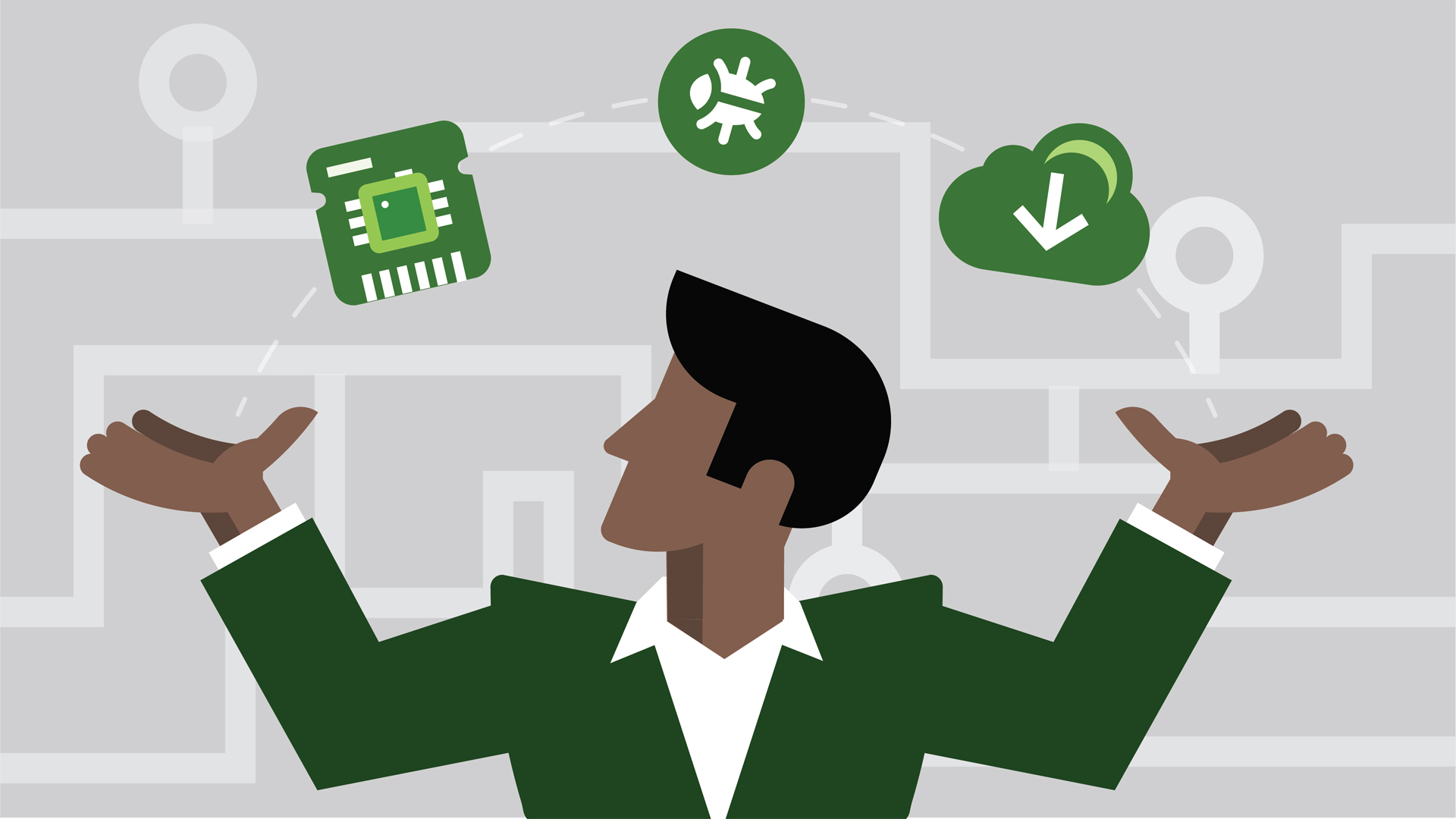
Function is nothing but a group of codes put together and given a name. And these can be called anytime without writing the whole code again and again. Functions make our code neat and easily readable and avoid us to rewrite the same code again and again. There are two types of functions in c, library functions and user defined functions. Library functions are pre-defined functions in C such as printf( ) and scanf( ) . User defined functions are functions that we, as programmers define.
Declaring and defining a function
Just like variables in C, we must declare and define a function. We declare a function in c by three components, return type, function name and parameters. The definition of a function is nothing more than the code block for the user defined function. When declaring a function, we must specify the parameter type along with the parameter. If the return type is not void, the function must have a return statement in the function definition.
float average( int num1, int num2 ) { float avg; avg = ( num1 + num2 ) / 2.0 ; return avg; }
Calling user defined functions
To use a function, we need to call it. Once we call a function, it performs its operations and after that the control again passes to the main program. To call a function, we need to specify the function name along with its parameters. we can call a function from inside another function or call it from itself ( recursion ). Calling a function in main( ) is no different than calling a function from another function is main is just another function.
Calling a function from another function
float average( int num1, int num2 ) { float avg; avg = ( num1 + num2 ) / 2.0 ; return avg; } int main() { float avg = 0.0 ; avg = average( 6, 12 ) ; printf("%2.1f", avg ) ; return 0; }
Will output 6.0
Recursion
#includeint factorial( int a ) { if( a == 0 || a == 1) { return 1; } else { return a*factorial(a-1); } } int main() { int n; n = 5 ; int fact = factorial(n); printf("Factorial of %d is %d ", n, fact); return 0; }
Will output Factorial of 5 is 120