Variables in C
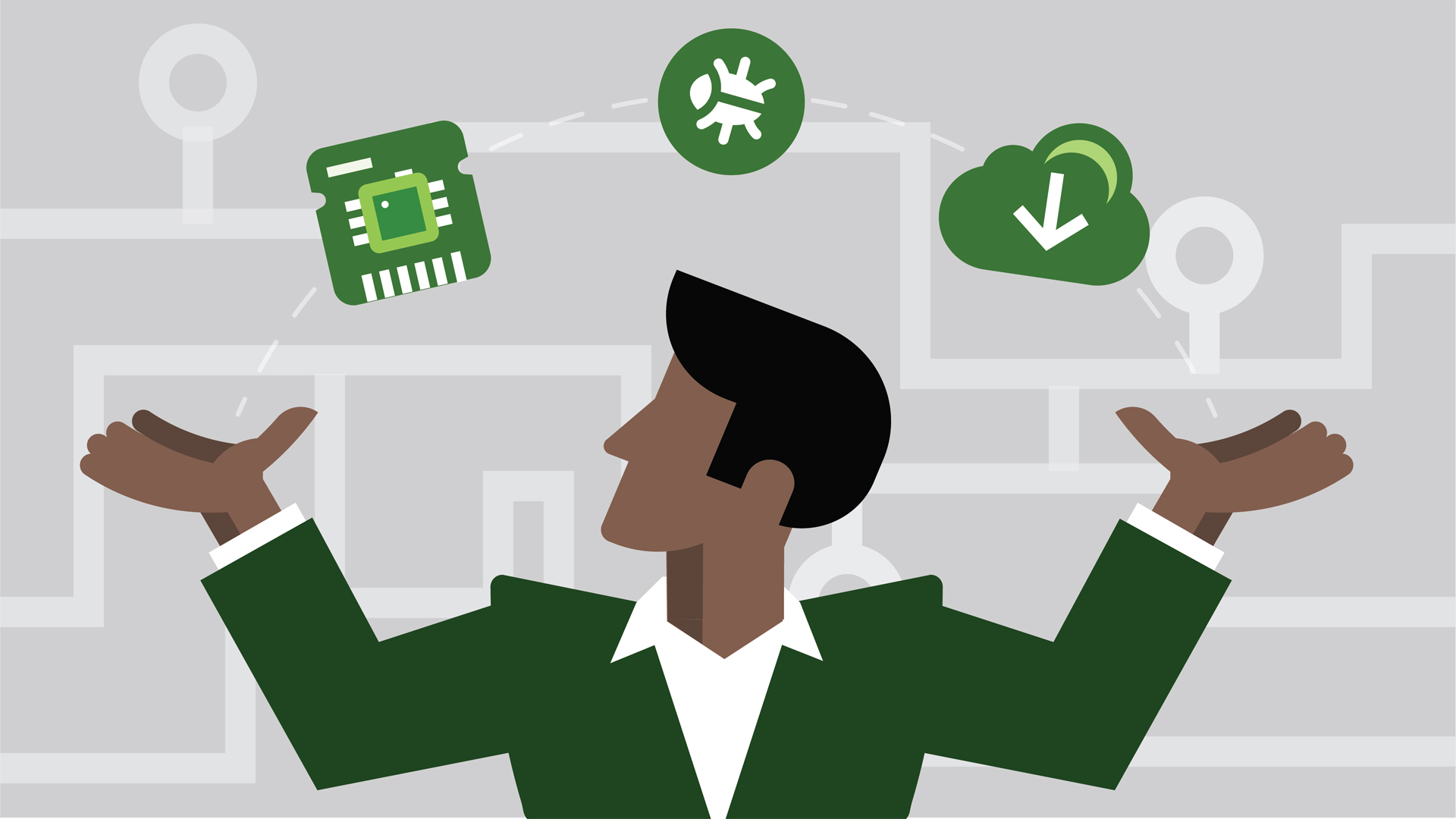
C is a general-purpose, procedural, computer programming language developed in 1972 by Dennis M. Ritchie at the Bell Telephone Laboratories to develop the UNIX operating system. C is the most widely used computer language. It keeps fluctuating at number one scale of popularity along with Java programming language, which is also equally popular and most widely used among modern software programmers.
A variable is a name given to a memory area that our programs can manipulate. Each variable in C has a specific type, which determines the range of values that can be stored within that memory and the set of operations that can be applied to the variable.
Defining a variable
A variable definition tells the compiler where and how much storage to create for the variable. A variable definition specifies a data type and contains a list of one or more variables of that type. There are some rules to defining a variable. Variable names can't start with a digit. Variable names can have special characters like underscore. Blank spaces are not allowed in variable names. Keywords aren't allowed in variable names. Variable names are case sensitive.
int a, b A ; char foo1 ; float item_cost ; double largeNumber ;
Declaring variables
Variables must be declared before they can be used used in the program. Declaration tells the compiler what the variable name is. It tells the compiler what data type the variable will hold. Declaring a variable means that we must initialize it. If most cases, if we attempt to use a variable without initializing it to some value, it will result in undefined behavior or the compiler may throw an error. If we make the variable static, outside the main code block, the compiler will set the value to 0 or null.
1 #include2 3 extern int num1, num2; 4 int c; 5 6 int main () 7 { 8 9 int d, e; 10 11 a = 7; 12 b = 14; 13 14 print f("%d ", c ) ; 15 c = a + b; 16 17 printf(": %d ", c); 18 c = num1 + num2 + b ; 19 printf(": %d", c ) ; 20 return 0; }
The above code will output 0 : 21 : 14