Inheritance in Java
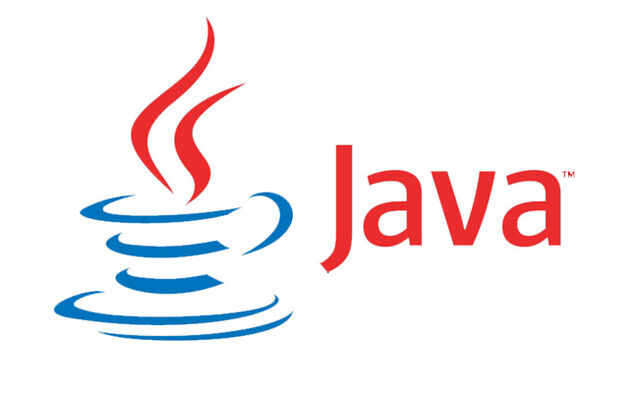
Inheritance is defined as the process where one class gets the properties of another class. Inheritance makes code more manageable in a hierarchical order. The class which inherits the properties of other is known as subclass, derived class, or child class and the class whose properties are inherited is known as superclass, base class, or parent class.
The idea of inheritance is simple but powerful. When we want to create a new class and there is already a class that includes some of the code that we want, we can derive our new class from the existing class. In doing this, we can reuse the fields and methods of the existing class without having to write them again. A subclass inherits all the members ( fields, methods, and nested classes ) from it's superclass. Constructors are not members, so they are not inherited by subclasses, but the constructor of the superclass can be invoked from the subclass.
Java uses the keyword extends to indicate that we are making a new class that derives from an existing class. The meaning of extends is to increase the functionality. In Java, a class which is inherited is called a parent or superclass, and the new class is called child or subclass. The super keyword is similar to this keyword. These keywords are used to differentiate the members of superclass from the members of subclass, if they have same names. The super keyword is also used to invoke the superclass constructor from subclass. We use the super and this keyword to differentiated member of the superclass that have the same names as the sub class. Java supports three different types of inheritance, single, multilevel, and hierarchical. Java does not support multiple inheritance but we can achieve multiple inheritance only through interfaces.
Single and hierarchical inheritance
In single inheritance, subclasses inherit the features of one superclass. In Hierarchical Inheritance, one class serves as a superclass (base class) for more than one sub class. In both cases, the child(ren) all inherit from a single parent. In the case of single inheritance, the child has access to the methods of the parent class as well it's own. In the case of hierarchical inheritance, the same applies but the children don't have access to other child methods.
Single Inheritance
class Car { public void start() { System.out.println("Engine started"); } } class Sedan extends Car { public void doors() { System.out.println("I have 4 doors"); } } Public class Main { public static void main(String args[]) { Sedan myCarInstance = new Sedan(); myCarInstance.start(); myCarInstance.doors(); } }
Hierarchical Inheritance
class Car { public void start() { System.out.println("Engine started"); } } class Sedan extends Car { public void doors() { System.out.println("I have 4 doors"); } public void trunk() { System.out.println("trunk opened"); } } class Coupe extends Car { public void doors() { System.out.println("I have 2 doors"); } public void hatch() { System.out.println("hatch opened"); } } Public class Main { public static void main(String args[]) { Sedan mySedan = new Sedan(); Coupe myCoupe = new Coupe(); mySedan.start(); mySedan.doors(); mySedan.trunk(); myCoupe.start(); myCoupe.doors(); myCoupe.hatch(); } }
Multilevel inheritance
In Multilevel Inheritance, a derived class will be inheriting a base class and as well as the derived class also act as the base class to other class. In Java a class cannot directly access the grandparent's members.
class Car { public void start() { System.out.println("Engine started"); } } class Sedan extends Car { public void doors() { System.out.println("I have 4 doors"); } } class Lemon extends Sedan { public void fix() { System.out.println("I need to be fixed"); } } Public class Main { public static void main(String args[]) { Lemon myLemon = new Lemon(); myLemon.start(); myLemon.doors(); myLemon.fix(); } }
Multiple Inheritance through Interface
In Multiple inheritance ,one class can have more than one superclass and inherit features from all parent classes. Please note that Java does not support multiple inheritance with classes. In java, we can achieve multiple inheritance only through Interfaces.
interface sedan { public void doors() { } } interface lemon { public void fix() { } } interface car extends sedan, lemon { public void start() { } } public class Automobile implements car { public void fix() { System.out.println("Fix Me"); } public void start() { System.out.println("engine started"); } public void doors() { System.out.println("I have 4 doors"); } } Public class Main { public static void main(String args[]) { Automobile myAuto = new Automobile(); myAuto.start(); myAuto.doors(); myAuto.fix(); } }