Classes and objects in Java
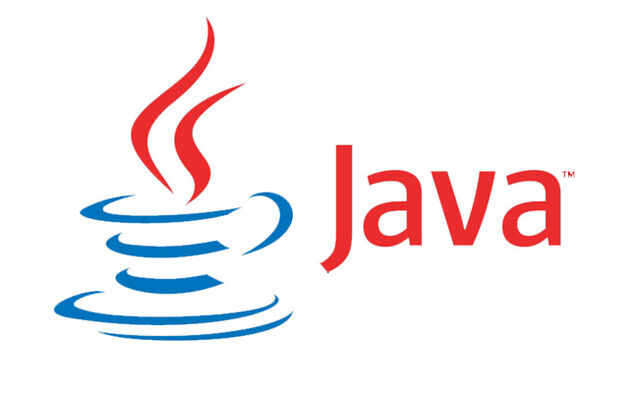
Java is an Object-Oriented Language which is a software design method that models the characteristics of real or abstract objects using software classes and objects. An object represents real entities and consists of state, behavior and identity. A class is a user defined template or prototype from which objects are created. It is the set of properties and / or methods that are common to all objects of one type.
Class
Basic classes consists of modifiers, name, and body. The modifier affects its runtime behavior. The class name, as the title implies, is the name of the class and begins with a letter. The body is the part surrounded by {}. Classes can contain any one of the following variable types, local, instance and class. Local variable are variables defined inside methods and the variable scope is only in the method so the variable is gone when the method is finished. Instance variables are variables in a class but outside any method in the class. Instance variables are initialized when the class is instantiated. Class variables are declared in a class but outside any method and use the static keyword.
Since classes are templates for creating objects, class definitions will need to have a constructor. Constructors are used for initializing new objects. Fields are variables that provides the state of the class and its objects, and methods are used to implement the behavior of the class and its objects.
1 public class Car 2 { 3 String make ; 4 String model ; 5 6 public Car() 7 { 8 } 9 10 public Car(String type) 11 { 12 12 } 14 void accelerate() 15 { 16 Boolean isStarted ; 17 } 18 19 }
Lines 3 and 4 are instance variables.
Lines 6 and 10 are constructors for the class.
Line 14 is a class method.
Object
Objects has state, behavior and identity. State is the attributes of an object, it has the properties of an object. Behavior is the methods of an object, what the object does. Identity is the unique name of an object and enables the object to interact with other objects. There are three steps involved when creating an object from a class; declaration, instantiation and initialization. We declare objects by giving a variable name to an object type. The object type, which is the class name. Instantiation and initialization creates the new object is uses the keyword new.
public class Car { public Car(String owner) { System.out.println("Car owner is " + owner ); } public static void main(String []args) { Car myCar = new Car( "John" ); } }
Will print "Car owner is John".
Accessing instance variables and methods
Instance variables and methods are accessed via created objects. To access an instance variable, we use the object name followed by a . followed by the instance variable or method. If the method has parameters, the parameters must be passed also. In order to access the methods and instance variables, they must not be declared using the private keyword. Below some examples of accessing instance variables and methods.
1 public class Car 2 { 3 int numDoors ; 4 5 public Car(String owner) 6 { 7 System.out.println("Owner is :" + owner ); 8 } 9 10 public void setNumDoors( int doors ) 11 { 12 numDoors = doors; 13 } 14 15 public int getDoors( ) 16 { 17 System.out.println("Car has " + numDoors + " doors" ); 18 return numDoors; 19 } 20 21 public static void main(String []args) 22 { 23 24 Car myCar = new Car( "John" ); 25 26 27 myCar.setNumDoors( 2 ); 28 29 myCar.getDoors( ); 30 31 System.out.println("Variable Value :" + myCar.numDoors ); 32 } 33 }