File operations in Python
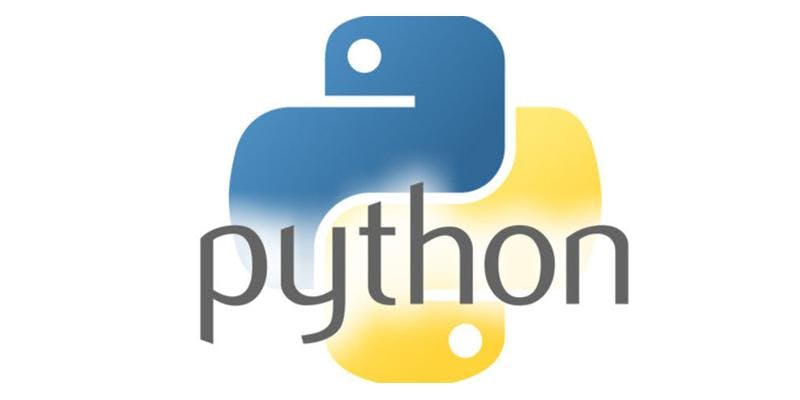
When working with Python, there is no need to import a library to read and write files. It is handled natively in the language similar to PHP. In some languages such as Java, we must import classes in order to write to a file, such as FileWriter. The first thing we need to do is use Python's built-in open function to get a file object. The open function opens a file. When you use the open function, it returns something called a file object or file handler. File objects has methods and attributes that can be used to collect information about the file that was opened. They can also be used to manipulate the file. The mode attribute of a file object tells us which mode a file was opened in. And the name attribute tells us the name of the file that the file object has opened. In Python, there are 4 main, read, write, append and read/write. In other languages such as PHP, there are variations of the 4 modes having to do with where the file object is pointing to the beginning of the file or at the end of the file. Below are the file object modes :
'r' Read only mode which is used when the file is only being read 'w' Write only mode which is used to edit and write new information to the file Any existing files with the same name will be erased when this mode 'a' Appending mode, which is used to add new data to the end of the file 'x' Creates a new file. If file already exists, the operation fails. '+' Read and write mode, which is used to handle both actions when working with a file 't' Opens the file in text mode 'b' Opens the file in binary mode.
Reading from a file
To open a file, Python uses the following syntax :
file_object = open( FILE_NAME, OPEN_MODE )
Python, as well as other languages such as Java and PHP, differentiated between text files and binary files. To open a text file in Python or PHP, we can use the 't' mode flag or not include it since it is the default mode. To open a binary file in Python or PHP, we must use the 'b' flag to tell the file object that this is a binary file and the language won't translate the data in the file. In Java, however, we must import a different set of libraries such as FileInputStream and FileOutputStream.
To read from a text file, we will need to create a file object using the open method then use the read method to read the data from the file. In Python, we can use the read() method in two ways. If we want to read the whole file, we don't include any parameters to the read method. If we want to read only a certain number of characters from a file, we would include the number of characters that we want to read. This is similar to PHP. To read a single line, both Python and PHP has a method to do so. In Python, we use the readline() where as in PHP, the equivalent of readline is fgets( ).
1 file_object = open( "foo.txt", 'r') 2 3 print file_object.read( ) 4 print file_object.read( 5 ) 5 print file_object.readline( )
Writing to a file
To write to a file, we will use the Python write() method. In Python in order to write something other than a string, it needs to be converted to a string first. In PHP, numbers don't have to be converted to strings.
1 file_object = open( "foo.txt", 'w') 2 3 print file_object.write("Hello World" ) 4 print file_object.write( str(5) )
Remember to close the file object
Once we are done with all the file input / output operations that we need to do, we must close the file object. To do so, we would use the close() method. Like Java and PHP, while the file can only have one file object at a time pointing to it. Here is some code to illustrate the need to close the file.
1 file_object = open( "foo.txt", 'w') 2 3 print file_object.write("Hello World" ) 4 5 file_object2 = open( "foo.txt", "w" ) 6 print file_object2.write("Good Bye") 7 file_object.close() 8 file_object2.close()
The code above will produce a file that has "Hello World" not "Good Bye."
1 file_object = open( "foo.txt", 'w') 2 3 print file_object.write("Hello World" ) 4 5 file_object.close() 5 file_object2 = open( "foo.txt", "w" ) 6 print file_object2.write("Good Bye") 7 file_object2.close()
The code above will produce a file that has "Good Bye."