Sort dictionary
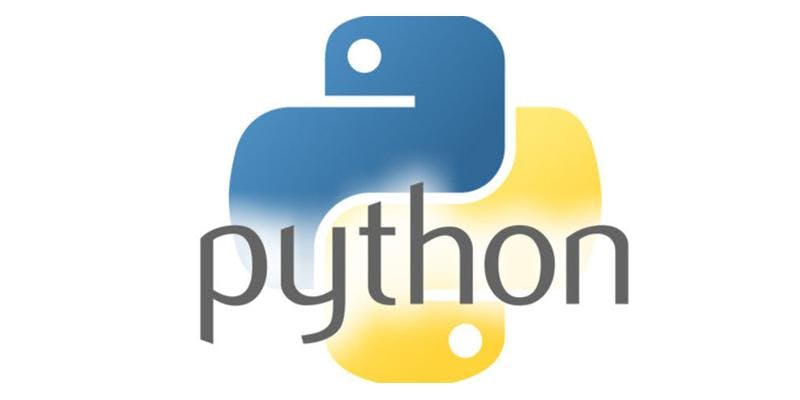
Sometimes you might need use a dictionary but still be able to sort it by value. There are many ways to accomplish this task and I would like to discuss one of the ways we are using here at Walden Systems, because we believe this is the most straightforward method to sort a dictionary. We will be using the OrderedDict type which keeps items in the order in which they were added.
Strategy to sort a dictionary is fairly simple: First, we need to iterate through the dictionary in sorted order using one of the built in methods. Second, we need to create a new ordered dictionary to store the the sorted items. Third, we need to add items to the new, ordered dictionary in sorted order.
Here are actual code samples:
The first thing you need to do is import the OrderedDict library with the following line
from collections import OrderedDict
Then you create a blank, OrderedDict with the following line :
sorted_dict = OrderedDict( )
Then you iterate through a dict in sorted order by value with the following line :
for l_key, l_value in sorted( p_dict.iteritems( ), key = lambda( key, value ) : ( value, key ) ) :
Finally, you will need add the items one by one into the OrderedDict with the following line :
sorted_dict[ l_key ] = l_value
So, this is how a final code for sorting a dictionary :
1 from collections import OrderedDict 2 3 sorted_dict = OrderedDict( ) 4 for l_key, l_value in sorted( p_dict.iteritems( ), key = lambda( key, value ) : ( value, key ) ) : 5 sorted_dit[ l_key ] = l_value