Sorting an array, case insensitive
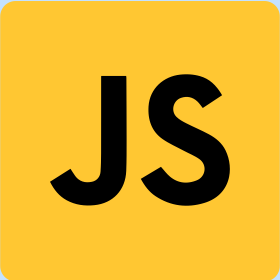
Sometimes you might need to sort an array in JavaScript that is case insensitive. There are many ways to accomplish this task and I would like to discuss one of the ways we are using here at Walden Systems, because we believe this is the most straightforward method to sort an array.
Strategy to sorting an array is straight forward and involves only two steps: First, we will use is the built in JavaScript function "sort( )". Second, we need to create a function using the built in JavaScript function "toLowerCase()" and "localeCompare()".
Here are the steps:
First thing we will use is the built in function sort :
SomeArray = [ "Mary", "had", "a", "Little", "lamb" ] ; SomeArray.sort() ;
Next, we will create a function to put inside the built in function sort(). We will need to use the toLowerCase( ) function to convert the string to lower case and will use the localeCompare( ) function to do the actual comparison:
function ( a, b ) { return a.toLowerCase( ).localeCompare(b.toLowerCase( ) ) ; }
When we put them together, we get the case insensitive sort:
SomeArray = [ "Mary", "had", "a", "Little", "lamb" ] ; SomeArray.sort(function (a, b) { return a.toLowerCase( ).localeCompare(b.toLowerCase( ) ) ; } ) ;
which will give the the following:
a had lamb Little Mary