How to write to a file in java using FileOutputStream
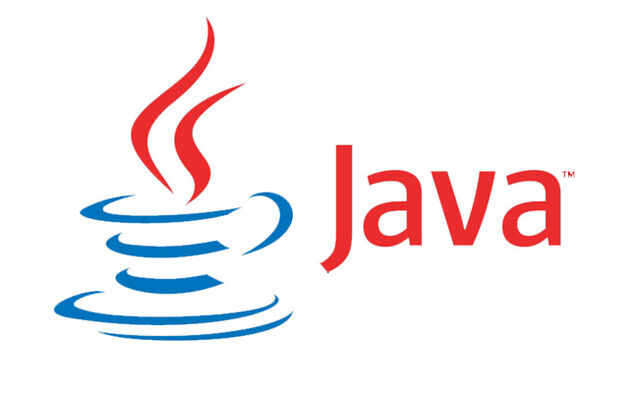
Java Powers the Biggest Technology. Mobile. Web apps. The Internet of Things. Big data. Machine learning. Cloud platform and cloud infrastructure. The next big things are here now, and Java is at the center of them all. Whether you’re developing the robust enterprise back end, building the agile front end, or thriving in a DevOps role, Java skills can up your game. With more than 10 million programmers and 13 billion Java-enabled devices worldwide, Java is the language that connects applications to data, data to people, and people to their digital lifestyle.
we will see how to write to a file in java using FileOutputStream. We would be using write() method of FileOutputStream to write the content to the specified file. Here is the signature of write() method.
1 public void write(byte[] b) throws IOException
It writes b.length bytes from the specified byte array to this file output stream. As you can see this method needs array of bytes in order to write them into a file. Hence we would need to convert our content into array of bytes before writing it into the file.
In the following example, we are writing a String to a file. To convert the String into an array of bytes, we are using getBytes() method of String class
1 import java.io.File; 2 import java.io.FileOutputStream; 3 import java.io.IOException; 4 5 public class WriteFile 6 { 7 public static void main(String[] args) 8 { 9 FileOutputStream fos = null; 10 File file; 11 String mycontent = "The quick brown fox jumped over the " + 12 "lazy moon"; 13 try 14 { 15 //Specify the file path here 16 file = new File("C:/foo.txt"); 17 fos = new FileOutputStream(file); 18 19 /* Checks whether the file 20 * exists or not. If the file is not found 21 * at the specified location it would create 22 * a new file*/ 23 if (!file.exists()) 24 { 25 file.createNewFile(); 26 } 27 28 /*Convert string to bytes so it can be wriiten to 29 * a file. 30 */ 31 byte[] bytesArray = mycontent.getBytes(); 32 33 fos.write(bytesArray); 34 fos.flush(); 35 System.out.println("File created"); 36 } 37 catch (IOException ioe) 38 { 39 ioe.printStackTrace(); 40 } 41 finally 42 { 43 try 44 { 45 if (fos != null) 46 { 47 fos.close(); 48 } 49 } 50 catch (IOException ioe) 51 { 52 System.out.println("Error in closing the Stream"); 53 } 54 } 55 } 56 }