Global and local scoping
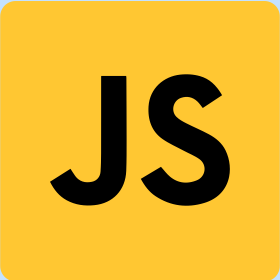
Understanding variable scope in JavaScript is one of the keys to building a solid foundation. The scope of a variable is controlled by the location of the variable declaration, and defines the part of the program where a particular variable is accessible. Scoping rules vary from language to language. To see how Python treats variable scoping, look here. To see how Bash treats variable scoping, look here. JavaScript has two scopes, global and local.
1 var f_name = "John"; 2 var lastName = function () 3 { 4 var lastName = "Doe"; 5 console.log( f_name ) ; 6 return l_Name ; 7 } 8 console.log( l_name ) ;
The variable "f_name" on line 1 is a global variable and can be accessed in the function block so the output of line 5 will be "John."
The variable "l_name" on line 4 is a local variable and can't be accessed outside the function block so line 8 will produce an uncaught ReferenceError: l_name is not defined.
Like Bash, any variable declared outside of a function belongs to the global scope, and is therefore accessible from anywhere in your code. Each function has its own scope, and any variable declared within that function is only accessible from that function and any nested functions. Because local scope in JavaScript is created by functions, it's also called function scope. When we put a function inside another function, then we create a nested scope. Currently, JavaScript, unlike many other languages, does not support block level scoping. This means that declaring a variable inside of a block structure like a for loop, does not restrict that variable to the loop. Instead, the variable will be accessible from the entire function.
1 var f_name = "John" ; 2 function myFunction () 3 { 4 m_name = "Q" ; 5 console.log( m_name ) ; 6 var innerFunction = function () 7 { 8 l_name= "Public" ; 9 console.log( l_name ) ; 10 return ( l_name ) ; 11 } 12 console.log( f_name ) ; 13 myFunction( ) ;
The above code will output the following lines :
   John
   Q
   Public
Variable shadowing
In JavaScript, variables with the same name can be specified at multiple layers of nested scope. In such case local variables gain priority over global variables. If you declare a local variable and a global variable with the same name, the local variable will take precedence when you use it inside a function. This type of behavior is called shadowing. Simply put, the inner variable shadows the outer.
1 var foo = "Hello" : 2 function writeFoo( foo ) 3 { 4 console.log( foo ) ; 5 } 6 console.log( foo ) ; 7 writeFoo( "World" ) ; 8 console.log( foo ) ;
The code above will have the following output :
   Hello
   World
   Hello
Local scope
When a JavaScript interpreter is trying to find a particular variable, it starts at the innermost scope being executed at the time, and continue until the first match is found, no matter whether there are other variables with the same name in the outer levels or not. It starts at the innermost scope being executed at the time, and continue until the first match is found, even if there are other variables with the same name in the outer levels.
1 var name = "John Doe" ; 2 function newScope( ) 3 { 4 name = "Jane Doe" ; 5 console.log( name ) ; 6 } 7 newScope( ) ; 8 console.log( name ) ;
The code above will have the following output :
   Jane Doe
   John Doe
Overwriting globally scoped variables in local functions
In the above example, even though both the local variable and the global variable had the same name, it didn't overwrite the global variable. But this is not always the case.
1 var foo ; 2 function myFunction () 3 { 4 foo = "first scope" ; 5 console.log( foo ) ; 6 var innerFunction = function () 7 { 8 foo = "second scope" ; 9 console.log( foo ) ; 10 }; 11 console.log( foo ) ; 12 }; 13 console.log( foo ) ; 14 myFunction( ) ; 15 console.log( foo ) ;
The code above will have the following output :
   undefined
   first scope
   second scope
   second scope
   second scope
This time the local variable test overwrites the global variable with the same name. When we run the code inside myFunction() function the global variable is reassigned. If a local variable is assigned without first being declared with the var keyword, it becomes a global variable. To avoid such unwanted behavior you should always declare your local variables before you use them. Any variable declared with the var keyword inside of a function is a local variable