Variables
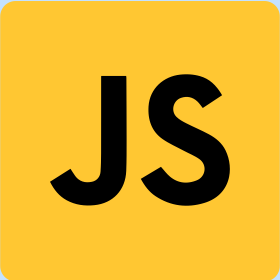
The variable is one of the most fundamental concepts in any programming language. The first step towards becoming proficient in JavaScript or any programming language is having a good understanding of variables. Variables can be a little confusing, especially to beginning programmers.
A variable is a way to store information so that you can later use it. A variable is a symbolic name that represents some data that you set. To think of a variable name in real world terms, picture that the name is a box and the data it represents are the contents of the box. The name wraps up the data so you can move it around a lot easier, but the name is not the data.
JavaScript is considered a "weakly typed" language because the data type of the variable doesn't have to be declared. In Javascript, like Python and BASH, data types of variables doesn't have to be declared and you can change the data type of the variable just by storing a different data type.
Before you can use a variable, you have to create it. In programming, creating a variable is called "declaring a variable". In JavaScript, you create a variable using a two step process: find a name for your variable and transform that name into a variable.
Finding a name
Before you can create a variable, you have to come up with a name for it. You can call it "x," "y," or any other name as long as you can remember it. It is often advisable to give a name which will allow you to easily recognize what the variable contains. A simple rule of thumb is to use a name which is descriptive of what you intend to store in the variable.
Transform the name to a variable
When choosing a variable name, you must choose a valid name. If the variable name isn't valid, you will receive a JavaScript error. To make sure that you choose a valid variable name, follow these basic rules:
The first character must be a letter or an underscore (_). You can't use a number as the first character. The rest of the variable name can include any letter, any number, or the underscore. You can't use any other characters, including spaces, symbols, and punctuation marks. As with the rest of JavaScript, variable names are case sensitive. That is, a variable named Interest_Rate is treated as an entirely different variable than one named interest_rate. There's no limit to the length of the variable name. You can't use one of JavaScript's reserved words as a variable name. All programming languages have a supply of words that are used internally by the language and that can't be used for variable names because doing so would cause confusion. ( see below for list of reserved word )
Reserved words
abstract else instanceof super boolean enum int switch break export interface synchronized byte extends let this case false long throw catch final native throws char finally new transient class float null true const for package try continue function private typeof debugger goto protected var default if public void delete implements return volatile do import short while double in static with
Transform the name into a variable
Once you have a name, you need to make it a variable. In JavaScript, you do this by using the keyword "var". The "var" keyword is used to declare a variable. As long as you place "var" to the left of any word, that word automatically becomes a variable. Below are some examples.
var first_name ; var last_name ; var age ;
Data types
Javascript has seve basic data types. Below is a list of the 7 data types:
number
Numbers of any kind: integer or floating-point.
string
Strings. A string may have one or more characters, there’s no separate single-character type.
boolean
True/false.
null
Unknown values – a standalone type that has a single value null.
undefined
Unassigned values – a standalone type that has a single value undefined.
object
Used for more complex data structures.
symbol for unique identifiers.
Variable scope
The scope of a variable is the region of your program in which it is defined. JavaScript variables have only two scopes:
Global Variables
A global variable has global scope which means it can be defined anywhere in your JavaScript code.
Local Variables
A local variable will be visible only within a function where it is defined. Function parameters are always local to that function.
Within the body of a function, a local variable takes precedence over a global variable with the same name. If you declare a local variable or function parameter with the same name as a global variable, you effectively hide the global variable.
Javascript type conversion
To get the data type of a variable, use the typeof operator. Here are some examples.
typeof "John"
Returns "string"
typeof 3.14
Returns "number"
typeof NaN
Returns "number" since the data type of NaN is number
typeof false
Returns "boolean"
typeof [1,2,3,4]
Returns "object" since the data type of an array is object
typeof {name:'John', age:21}
Returns "object"
typeof new Date()
Returns "object" since the data type of a date is object
typeof function () {}
Returns "function"
typeof myfoo
Returns "undefined" * since the data type of an undefined variable is undefined * and the data type of a variable that has not been assigned a value is also undefined *
typeof null
Returns "object" since the data type of null is object