Dictionary
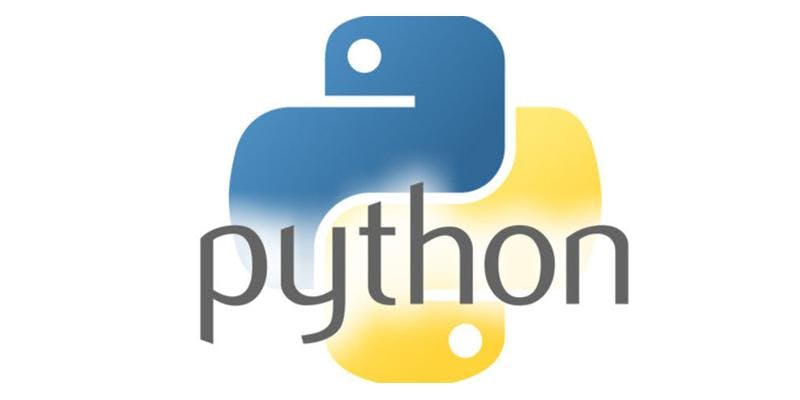
A dictionary is composite data type which is similar to a list in that it is a collection of objects. A dictionary is a collection which is unordered, changeable and indexed. Each key is separated from its value by a colon ( : ), the items are separated by commas, and is enclosed in curly braces. An empty dictionary without any items is written with just two curly braces, like this: {}. Keys must be unique within a dictionary but values doesn't have to be unique. The values of a dictionary can be of any type, but the keys must be of an immutable data type such as strings, numbers, or tuples.
Getting values in a dictionary
To access dictionary elements, you use the square brackets along with the key to obtain its value. If you try to access a data item with a key that is not part of the dictionary, you get an error.
dict = {'FName': 'John', 'LName':'Doe', 'Age': 21 } print dict['FName']
The code above outputs John
dict = {'FName': 'John', 'LName':'Doe', 'Age': 21 } print dict['John']
The above code outputs the following error
Traceback (most recent call last): print dict['John']; KeyError: 'John'
Updating dictionary
You can update a dictionary by adding a new key-value pair, modifying an existing key value pair, or deleting an existing key value pair. You can either remove individual dictionary elements or clear the entire contents of a dictionary. You can remove the entire dictionary by using the del statement.
dict = {'FName': 'John', 'LName':'Doe', 'Age': 21 } del dict['FName']
The above code will remove the "FName key value pair and dict will now hold {'LName':'Doe', 'Age': 21}
dict = {'FName': 'John', 'LName':'Doe', 'Age': 21 } dict.clear( )
The above code will remove all the key value pairs and dict will now be empty
dict = {'FName': 'John', 'LName':'Doe', 'Age': 21 } del dict
The above code will delete the dictionary, dict and dict will no longer exist
Dictionary key properties
Dictionary values have no restrictions. They can be any arbitrary Python object, either standard objects or user-defined objects. Keys, on the other hand, have 2 rules. The first rule is that than no duplicate keys are allowed. When duplicate keys encountered during assignment, the last key value pair used gets the assignment.
The second rule is that keys must be immutable. This means that you can use strings, numbers and tuples as keys.