Strings in Python
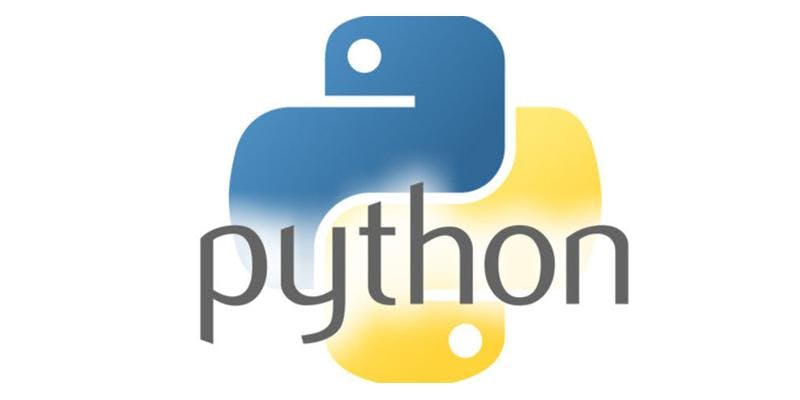
Strings the most common type in Python. Strings are considered an aggregate type, other aggregate types include tuples and lists. We can create them by enclosing characters in quotes. We can use either single quotes or double quotes since python treats single quotes in a similar way to double quotes. Creating strings is as simple as assigning a value to a variable. Python does not support a character types so characters are treated as strings of length one and are also considered a substring. To access substrings, use the square brackets for slicing along with the index or indices to obtain your substring. To update strings, you just have to reassigning a variable to a new value.
Declaring Python strings
str1 = "Hello World"
str2 = 'Hello World'
Substrings
str1 = "Hello World"
substr1 = str1[ 0:4 ]
substr2 = str1[ 0 ]
substr1 has the value "Hello" and substr2 has the value "H"
Updating strings
str1 = "Hello World"
str1 = "Good morning"
str1 holds the value "Good morning"
Python has a list of non printable characters or escape characters that are represented with a backslash. Below is a list of special, escape characters.
Escape characters
a bell or alert backspace Cx [Control] x cx [Control]x e escape f formfeed new line nn octal notation s space carriage return tab v vertical tab xnn Hexadecimal notation
String operators
There are many operations that can be performed with string which makes it one of the most used datatypes in Python. Below are some of the string operators available.
One of the string operators, the format operator ( % ), is unique to strings and has several sub operators of their own.
br>
For the operators listed below, we will assume the following:
str1 = "Hello"
str2 = "World"
String Operators
Concatenate joins two strings + str2 + str2 holds "HelloWorld" Repetition concatenates copies of string * str1*2 holds "HelloHello" Slice gives the character from the given index [] str[0] returns "H" Range slice gives the characters from the given range [ : ] str[0:3] returns "Hell" Membership returns true if character exists in string in "H" in str1 returns true Not membership returns true if character doesn't exist not in "H" not in str1 returns false Format performs string formatting %
Formatting
Convert to character %c Convert to signed decimal integer %i Convert to unsigned decimal integer %u Convert to octal integer %o Convert to Hexadecimal lowercase %x Convert to exponential notation %e Convert to floating point %f
Built in string methods
capitalize()
Capitalizes first letter of string
center(width, fillchar)
Returns a space-padded string with the original string centered to a total of width columns.
endswith(suffix, beg=0, end=len(string))
Determines if string or a substring of string (if starting index beg and ending index end are given) ends with suffix; returns true if so and false otherwise.
expandtabs(tabsize=8)
Expands tabs in string to multiple spaces; defaults to 8 spaces per tab if tabsize not provided.
find(str, begin=0 end=len(string))
Determine if str occurs in string or in a substring of string if starting index beg and ending index end are given returns index if found and -1 otherwise.
rfind(str, beg=0,end=len(string))
Does the same thing as find(), but search backwards in string.
index(str, beg=0, end=len(string))
Does the same thing as find(), but raises an exception if str not found.
count(str, begin= 0,end=len(string))
Counts how many times str occurs in string or in a substring of string if starting index begin and ending index end are given.
decode(encoding='UTF-8',errors='strict')
Decodes the string using the codec registered for encoding. encoding defaults to the default string encoding.
encode(encoding='UTF-8',errors='strict')
Returns encoded string version of string; on error, default is to raise a ValueError unless errors is given with 'ignore' or 'replace'.
isalnum()
Returns true if string has at least 1 character and all characters are alphanumeric and false otherwise.
rindex( str, beg=0, end=len(string))
Does the same thing as index(), but search backwards in string.
isalpha()
Returns true if string has at least 1 character and all characters are alphabetic and false otherwise.
isdigit()
Returns true if string contains only digits and false otherwise.
islower()
Returns true if string has at least 1 cased character and all cased characters are in lowercase and false otherwise.
isnumeric()
Returns true if a unicode string contains only numeric characters and false otherwise.
isspace()
Returns true if string contains only whitespace characters and false otherwise.
istitle()
Returns true if string is properly "titlecased" and false otherwise.
isupper()
Returns true if string has at least one cased character and all cased characters are in uppercase and false otherwise.
len(string)
Returns the length of the string
join(seq)
Merges the string representations of elements in sequence seq into a string, with separator string.
ljust(width[, fillchar])
Returns a padded string with the original string left-justified to a total of width columns.
rjust(width,[, fillchar])
Returns a padded string with the original string right-justified to a total of width columns.
lower()
Converts all uppercase letters in string to lowercase.
lstrip()
Removes all the whitespace in the beginning of the string.
rstrip()
Removes all trailing whitespace of string.
strip([chars])
Removes all the beginning whitespace and trailing whitespace of a string string.
max(str)
Returns the max alphabetical character from the string str.
min(str)
Returns the min alphabetical character from the string str.
replace(old, new [, max])
Replaces all occurrences of old in string with new or at most max occurrences if max given.