How to read a file
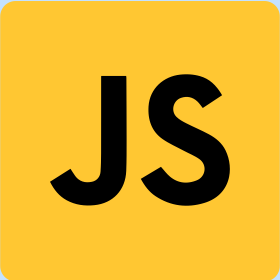
Sometimes you might need to open and read a file. There are many ways to accomplish this task and I would like to discuss one of the ways we are using here at Walden Systems, because we believe this is the most straight forward method to opening and reading a file.
Strategy to opening and reading a file is straight forward and involves only two steps: First, we will need to include the File System module. Next, we need to read the file using the readFile( ) method.
Here are the steps:
First thing we include the File System module :
var fs = require( 'fs' ) ;
Next, we need to open the file and read it's contents using the readFile( ) method. In this example, we will be opening a text file so we are using "utf8" as the return type:
fs.readFile
(
SOME_FILE, "utf8", function ( error, data )
{
if ( error )
{
throw error ;
}
console.log( data ) ;
}
) ;
So here is the full code :
var fs = require( 'fs' ) ;
fs.readFile
(
SOME_FILE, "utf8", function ( error, data )
{
if ( error )
{
throw error ;
}
console.log( data ) ;
}
) ;